Я понимаю, что это довольно длинный вопрос, но я не нашел здесь другого пути, кроме как опубликовать свой код, который я старался сделать максимально коротким и простым для ясности. Конечно, тонны лучших практик нарушаются, пример достаточно длинный, как есть.
Я сделал очень простое wpf-приложение, которое
- показывает список людей в левой части экрана (формат: имя и возраст между ())
- показывает все свойства выбранного человека в правой части экрана
- справа вы можете редактировать свойства и просматривать весь выбор в сообщении
В следующем примере я отредактировал возраст Бар. Однако в списке возраст не обновляется. Если я спрашиваю основную коллекцию, она все еще кажется обновленной ..
Как я могу сделать список знать?
Ниже приведены, помимо скриншота, код и XAML
ПРИМЕЧАНИЕ. Если изображение не отображается, попробуйте открыть его в новой вкладке или окне.
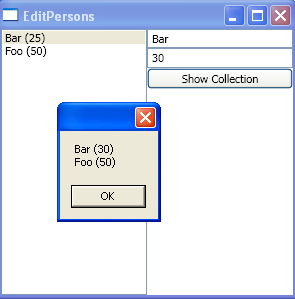
namespace ASAPBinding
{
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public override string ToString()
{
return String.Format("{0} ({1})",Name,Age);
}
}
}
namespace ASAPBinding
{
public class Dal
{
public ObservableCollection<Person> Persons { get; set; }
public Dal()
{
Persons = new ObservableCollection<Person>();
Persons.Add(new Person() {Name = "Bar", Age = 25});
Persons.Add(new Person() {Name = "Foo", Age = 50});
}
public void PrintOutCollection()
{
MessageBox.Show(
Persons[0].ToString() + "\n" + Persons[1].ToString()
);
}
}
}
<Window x:Class="ASAPBinding.EditPersons"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:ASAPBinding"
x:Name="window1"
Title="EditPersons" Height="300" Width="300">
<Window.Resources>
<local:Dal x:Key="dal"/>
</Window.Resources>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="1*" />
<ColumnDefinition Width="1*" />
</Grid.ColumnDefinitions>
<ListBox Name="ListBox1"
ItemsSource="{Binding Source={StaticResource dal}, Path=Persons, Mode=TwoWay}"
Grid.Column="0"/>
<StackPanel
DataContext="{Binding ElementName=ListBox1, Path=SelectedItem, Mode=TwoWay}"
Grid.Column="1" Margin="0,0,0,108">
<TextBox Text="{Binding Path=Name}" />
<TextBox Text="{Binding Path=Age}" />
<Button Click="Button_Click">Show Collection</Button>
</StackPanel>
</Grid>
</Window>
public partial class EditPersons : Window
{
public EditPersons()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
Dal dal = (Dal) window1.FindResource("dal");
dal.PrintOutCollection();
}
}