Причина этого (неправильного) поведения заключается в том, что на IOS13 представление UISearchBarBackground
больше не покрывает строку состояния. В предыдущих версиях IOS он действительно освещался.
IOS12 UISearchBarBackground
, охватывающий всю верхнюю область 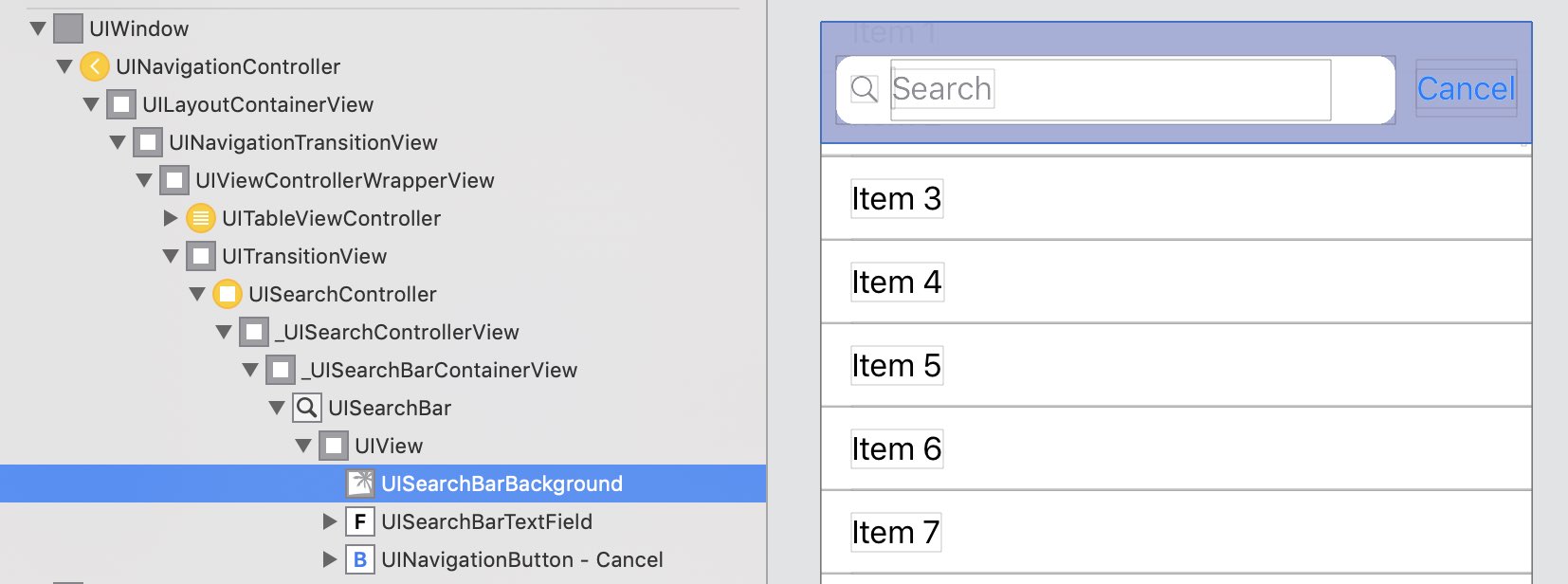
IOS13 UISearchBarBackground
, не покрывающий строку состояния 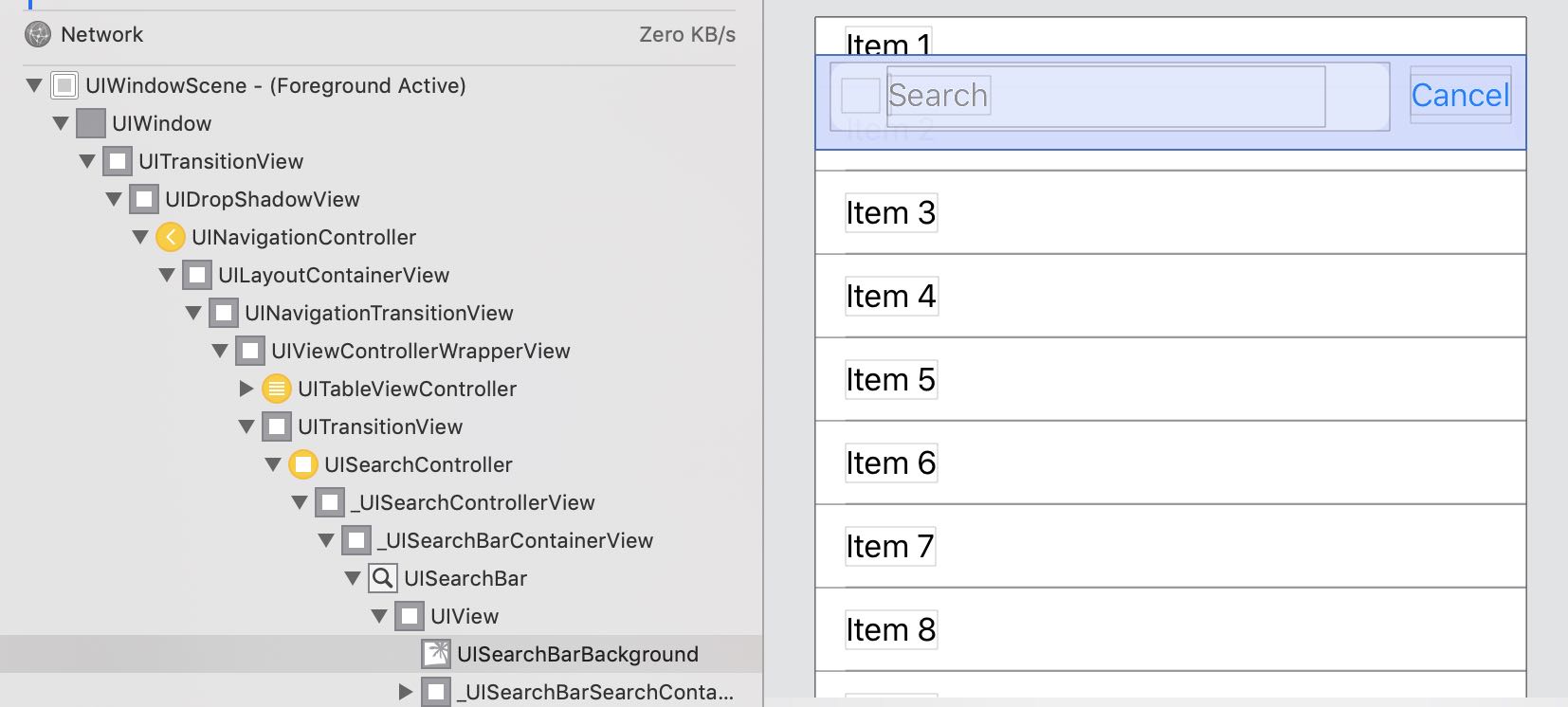
Решение, которое я придумал, не затрагивая другие иерархии ViewController в моем коде, было довольно неприятным хаком: расширение фрейма UISearchBarBackground
для соответствия состоянию в предыдущих версиях IOS13.
-(void)updateSearchResultsForSearchController:(UISearchController *)searchController {
NSString* searchText=searchController.searchBar.text;
if (@available(iOS 13.0, *)) {
if (searchController.active) {
//The intrinsic UISearchBar code seems to adjust the
//background view of UISearchBar later on thats why we
//need to delay
[self performSelector:@selector(adjustBg) withObject:nil afterDelay:0.1];
}
}
if (searchText.length==0) {
_filteredModel=_model;
} else {
NSPredicate *predicate = [NSPredicate predicateWithFormat:
@"SELF contains[c] %@",searchText];
_filteredModel=[_model filteredArrayUsingPredicate:predicate];
}
[_tc.tableView reloadData];
}
CGPoint pointInScreen(UIView* v,CGPoint pt)
{
CGPoint ptWin=[v.superview convertPoint:pt toView:nil];
CGPoint ptScreen=[v.window convertPoint:ptWin toWindow:nil];
return ptScreen;
}
-(void)adjustBg
{
//ugly: we try to adjust the background view of the UISearchBar
//to the top of the screen (like it was in pre IOS13 times)
if (!_search.active) {
return;
}
UISearchBar* bar=_search.searchBar;
if (bar.subviews.count==0) { return; }
UIView* first=bar.subviews[0];
if (first.class!=UIView.class || first.subviews.count==0) { return;}
UIView* bg=first.subviews[0];
if ([bg isKindOfClass:NSClassFromString(@"UISearchBarBackground")]) {
CGSize size=bg.frame.size;
CGPoint orig=bg.frame.origin;
CGPoint p=pointInScreen(bg,orig);
CGPoint p2=pointInScreen(bg, CGPointMake(0, orig.y+size.height));
if (p.y>0) { //not top of screen
bg.frame=CGRectMake(orig.x,-p.y,size.width,p2.y);
}
}
}
Это действительноне то решение, которое я искал, но пока нет лучшего, мне нужно сохранить его (и опубликовать здесь, если кто-то другой наткнется на это)