Опишите ошибку ?
Используя Google Maps API, я получаю указания и создаю DirectionsRenderer
. Все выглядит правильно, карта отображается, но маршрут не отображается, не уверен, что эта опция недоступна в GoogleMapReact
Воспроизвести ?
Шаги для воспроизведения поведения:
- Добавьте запрос на получение направлений в конструкторе
constructor(props: any){
super(props)
const DirectionsService = new google.maps.DirectionsService();
this.state = {};
DirectionsService.route(
{
origin: new google.maps.LatLng(19.5682414, -99.0436029),
destination: new google.maps.LatLng(19.7682414, -99.0436029),
travelMode: window.google.maps.TravelMode.DRIVING,
},
(result, status) => {
// console.log("status", status);
if (status === window.google.maps.DirectionsStatus.OK) {
this.setState({
directions: result,
});
console.log(result);
} else {
console.error(`error fetching directions ${result}`);
}
}
);
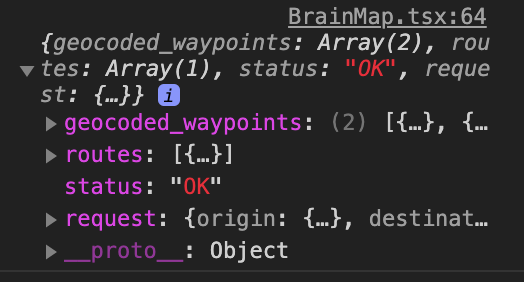
На этом этапе направления установлены в состоянии, поэтому давайте попробуем чтобы отобразить его.
render() {
return (
// Important! Always set the container height explicitly
<div id="BrainMap" className="schedulerMap justify-content-md-left">
<GoogleMapReact
bootstrapURLKeys={{ key: process.env.REACT_APP_MAPS_API_KEY }}
defaultCenter={this.initialPosition.center}
defaultZoom={this.initialPosition.zoom}
>
{this.state.directions && (
<DirectionsRenderer
directions={this.state.directions}
options={{
polylineOptions: {
strokeOpacity: 0.4,
strokeWeight: 4,
},
preserveViewport: true,
suppressMarkers: true,
}}
/>
)}
</GoogleMapReact>
</div>
);
}
Ожидаемое поведение ?
Я ожидаю увидеть маршрут, отображаемый на карте.
Другой контекст
В других примерах похоже, что они используют другой компонент GoogleMap и другие функции с составлением, я бы хотел избежать этого.
Код
import React, { Component } from "react";
import GoogleMapReact from "google-map-react";
import { DirectionsRenderer } from "react-google-maps";
import MapCard from "../commons/MapCard";
import "./Scheduler.css";
type BrainMapState = {
directions?: any;
}
class BrainMap extends Component {
state: BrainMapState;
initialPosition = {
center: {
lat: 19.4978,
lng: -99.1269,
},
zoom: 12,
};
constructor(props: any){
super(props)
const DirectionsService = new google.maps.DirectionsService();
this.state = {};
DirectionsService.route(
{
origin: new google.maps.LatLng(19.5682414, -99.0436029),
destination: new google.maps.LatLng(19.7682414, -99.0436029),
travelMode: window.google.maps.TravelMode.DRIVING,
},
(result, status) => {
// console.log("status", status);
if (status === window.google.maps.DirectionsStatus.OK) {
this.setState({
directions: result,
});
console.log(result);
} else {
console.error(`error fetching directions ${result}`);
}
}
);
//const directionsRenderer = new google.maps.DirectionsRenderer();
}
render() {
return (
// Important! Always set the container height explicitly
<div id="BrainMap" className="schedulerMap justify-content-md-left">
<GoogleMapReact
bootstrapURLKeys={{ key: process.env.REACT_APP_MAPS_API_KEY }}
defaultCenter={this.initialPosition.center}
defaultZoom={this.initialPosition.zoom}
>
{this.state.directions && (
<DirectionsRenderer
directions={this.state.directions}
options={{
polylineOptions: {
strokeOpacity: 0.4,
strokeWeight: 4,
},
preserveViewport: true,
suppressMarkers: true,
}}
/>
)}
</GoogleMapReact>
</div>
);
}
}
export default BrainMap;