Вы можете добавить FloatingActionButton
в RelativeLayout
в качестве родственного элемента RecyclerView
, отрегулировать его положение вверху и по центру родительского элемента.
Затем измените его backgroundTint
на ваш серый цвет (работает с API-21), ниже API-21, по умолчанию он принимает colorAccent
.
Добавить ic_baseline_arrow_upward_24.xml
из Android ресурсов в папку с возможностью переноса и использовать его в FAB android:src
атрибут.
Для прокрутки к началу RecyclerView
используйте scrollToPosition()
или smoothScrollToPosition()
FloatingActionButton fab = findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// recyclerView.scrollToPosition(0);
recyclerView.smoothScrollToPosition(0);
}
});
Макет:
<androidx.core.widget.NestedScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fillViewport="true"
tools:context=".Fragment.ImagesFragmentProject.WallpapersImagesFragment">
<!-- "descendantFocusability" to make the recyclerView not scrolls to the top itself -->
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:descendantFocusability="blocksDescendants">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView_image_wallpapers"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorBackground"
android:nestedScrollingEnabled="false"/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:background="#6D353232"
android:backgroundTint="#6D353232"
android:visibility="gone"
android:src="@drawable/ic_baseline_arrow_upward_24"
app:rippleColor="#6D353232" />
</RelativeLayout>
</androidx.core.widget.NestedScrollView>
Вы можно управлять, чтобы скрыть / показать кнопку fab, добавив пользовательские ReyclerView.OnScrollListener
и переопределив onScrolled()
и onScrollStateChanged()
методы
recyclerView.addOnScrollListener(new RecyclerView.OnScrollListener() {
@Override
public void onScrolled(@NonNull RecyclerView recyclerView, int dx, int dy) {
if (dy > 0) { // scrolling down
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
fab.setVisibility(View.GONE);
}
}, 2000); // delay of 2 seconds before hiding the fab
} else if (dy < 0) { // scrolling up
fab.setVisibility(View.VISIBLE);
}
}
@Override
public void onScrollStateChanged(@NonNull RecyclerView recyclerView, int newState) {
if (newState == RecyclerView.SCROLL_STATE_IDLE) { // No scrolling
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
fab.setVisibility(View.GONE);
}
}, 2000); // delay of 2 seconds before hiding the fab
}
}
});
Вот предварительный просмотр
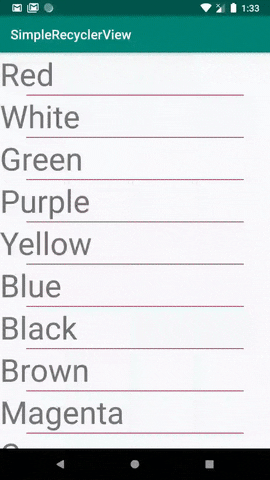